The array is frequently used in programming to store more than one data, this article is prepared to give information about the array in Swift.
Defination of Array
An array is a collection of similar data types. Arrays store data of similar data types, so you can access data of the same structure but different data in one place.
Let’s give an example: you are creating a school enrollment program and you will register 5 student names, it is easier to use 1 array than to create 5 variables.
Array stores each data in a separate block (no connection with other blocks) in this way, each block can be accessed with its own index number.
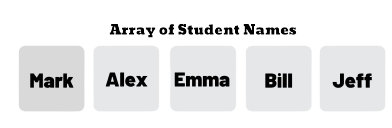
Indexes are specific to each block, with the help of an index, the data stored in that block can be accessed in the array.
Array Collection in Swift
Creating an array in Swift is simple. In the example below, an array containing numbers from 1 to 5 is created.
// array (it's just contain int)
var nums: [Int] = [1,2,3,4,5]
print("Array:" , (nums))
// Array: [1,2,3,4,5]
Swift is a type inference language, so it can determine what type an array is based on the data you add, so you don’t have to specify the data type.
var numbers = [1,2,3,4]
print("Array: ", (numbers))
// [1,2,3,4]
It is possible to create an empty array without entering data (you can enter data later with the help of functions.)
// Empty Int Array
var arr = [Int]()
The array we are currently creating does not contain any values, but it specifies that the values it will receive must be Int.
Empty arrays must be declared which data type to hold when creating since there are no auto-declarable because it’s doesn’t have value.
Access Value in Array
In Swift, each element in an array is associated with a number. The number is known as an array index.
There are two ways to access an item in an array in Swift, using a loop or indexing a single piece of data.
Note that indexes start at 0, the index number of the first element in an array is 0, not 1.
arr [String] = [10,20,30,40,50]
print(arr[0])
// 10
With the for loop, you can quickly access and modify all the elements in the array. It is possible to do the same in a while loop.
var foods = ["Sushi","Ramen","Burger"]
// Write all
for i in foods
{
print("Element:" , i);
}
// Change Elements
for i in 0...foods.count - 1
{
foods[i] = "New";
}
print("New Array": foods)
Element: Sushi
Element: Ramen
Element: Burger
New Array: ["New", "New", "New"]
Loops are easier and more preferable than indexing one by one. You can get more indexes from an array using ranges.
var foods = ["Sushi","Ramen","Burger"]
// ["Sushi", "Ramen", "Burger"]
print(foods[0...2])
Array Functions
We have a few ready-made array-specific functions to use arrays, these functions will make your work easier when working with arrays.
There are ready-made functions for many tasks such as adding, deleting, sorting, changing the index order, searching for the element, etc.
1 – Adding Element: Insert & Append
Swift, like many other languages, provides 2 functions for adding elements. Append adds an element to the end of the array, Insert adds it to the specified position.
// Append Example
var chars = ["x" , "y"]
chars.append("z")
print("Array:" , chars)
Array: ["x", "y", "z"]
You can incrementally fill an empty array one by one using append with loops. A while loop can be used instead of a for a loop.
// Append With Loop
var nums : [Int] = []
for item in 0...5
{
nums.append(item)
}
print(nums)
[0, 1, 2, 3, 4, 5]
Now let’s look at the insert function, where we can add a value to a certain location as opposed to appending.
// Add "x" in second index
var nums = ["x","z"]
nums.insert("y", at: 1)
print("Array:" , nums)
Array: ["x", "y", "z"]
2 – Remove Element
You can delete the elements in an array with the remove() function. The remove function takes 1 parameter, this parameter is the index number.
// Remove 4th element
var x = ["a","b","c","d","e","f"]
x.remove(at: 4)
print(x)
["a", "b", "c", "d", "f"]
There are 3 other functions similar to the remove function, although they are not used much (very useful in some places).
These remove functions are mostly used to use arrays in different ways or to preserve their operation in the program.
These functions are: removeFirst() , removeLast() and removeAll(): you can delete the first element, the last element, or all elements with these functions.
var nums: [Int] = [1,2,3,4,5]
print("Current:",(nums))
nums.removeFirst()
print("Current:",(nums))
nums.removeLast()
print("Current:",(nums))
nums.removeAll()
print("Current:",(nums))
Current: [1, 2, 3, 4, 5]
Current: [2, 3, 4, 5]
Current: [2, 3, 4]
Current: []
3 – Sorting Elements
if you don’t want to create your own sort function, but if you need fast sorting function, you can use the sort function by swift.
var nums = [1,4,3,5,9,7]
nums.sort()
print(nums)
[1, 3, 4, 5, 7, 9]
You can also use it to sort strings, it performs well, so it’s pretty handy if you don’t have an algorithm to customize based on data. Complexity O(n * log(n).
var id: [String] = ["b","q","a"]
id.sort()
print("Sorted Array:" , id)
Sorted Array: ["a", "b", "q"]
4 – Shuffle Function
The shuffle function is used to change the order of array elements. If change the order of the directories wouldn’t it be difficult for me to access them?
This question may be swirling in your mind right now, which is a perfectly reasonable theory, but that’s what you want anyway.
You can shuffle index orders in arrays to avoid accessing your data, so the attacker will have to deal with random indexes.
var nums = [1,2,3,4,5,6,7]
nums.shuffle()
print(nums)
[10, 3, 8, 7, 6, 4, 5]
5- Reverse Function
this function has a simple mission, reverses the order of array elements. You can use it if you need it.
var nums = [1,2,3,4,5,6,7]
nums.reverse()
print(nums)
[10, 9, 8, 7, 6, 5, 4]
Checking Empty Array
Being empty of an array can cause many errors, so you should use them more carefully.
There is an isEmpty variable that you can check if an array is empty. It tells you whether the array is full or not.
With the help of this variable, you can create many features such as preventing errors by automatically assigning default values to empty arrays.
// array
let numbers = [20 , 12 , 23 , 13]
// check if numbers is empty
var result = numbers.isEmpty
print("Array Empty:", result)
Array Empty: false
It returns a boolean value, it will return true if the array was empty, so you can use it in a conditional structure.
Using Array With Mixed Data Types (List)
If you’ve come from Python, you’ve probably heard of the list data type, which is similar to using an array with different data types.
Let’s give good news, the good news is that Swift lets you use lists, it’s really efficient when you need it.
To use an array with different data types, you must select the data type “Any” when creating the array.
“Any Data Type”: a special type in Swift that is used for working with non-specific types. It helps you store different types of data.
// Create New Array
var id: [Any] = ["Mark" , 001]
print("Name:", id[0])
print("Id:", id[1])
Name: Mark
Id: 1
You can include other data types such as boolean, float, and even add an object to this array.
Hope it helped – See you in other articles. If you want to share the article, please indicate the source.